We will learn how to automate Docker builds using Jenkins. We will use Java based application. I have already created a repo with source code +
Dockerfile. The repo also have Jenkinsfile for automating the following:
- Automating builds
- Automating Docker image creation
- Automating Docker image upload
- Automating Docker container provisioning
Pre-requisites:
1. Jenkins is up and running
2. Docker installed on Jenkins instance. Click to here to know how to integrate Jenkins and Docker.
3. Docker plug-in installed in Jenkins
4. user account setup in https://cloud.docker.com
- Automating builds
- Automating Docker image creation
- Automating Docker image upload
- Automating Docker container provisioning
Pre-requisites:
1. Jenkins is up and running
2. Docker installed on Jenkins instance. Click to here to know how to integrate Jenkins and Docker.
3. Docker plug-in installed in Jenkins
4. user account setup in https://cloud.docker.com
5. Port 8085 open in security firewall rules
Step #1 - Create Credentials for Docker Hub
Go to your Jenkins where you have installed Docker as well. Go to credentials -->
Click on Global credentials
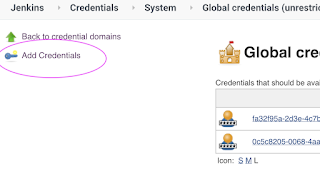
Now Create an entry for Docker Hub credentials
Make sure you take note of the ID as circled below:
Step # 2 - Create a scripted pipeline in Jenkins, name can be anything
Step # 3 - Copy the pipeline code from below
Make sure you change red highlighted values below:
Your docker user id should be updated.
your registry credentials ID from Jenkins from step # 1 should be copied
node {
def image
def mvnHome = tool 'Maven3'
stage ('checkout') {
checkout([$class: 'GitSCM', branches: [[name: '*/master']], doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [], userRemoteConfigs: [[credentialsId: '', url: 'https://bitbucket.org/ananthkannan/myawesomeangularapprepo/']]])
}
stage ('Build') {
sh 'mvn -f MyAwesomeApp/pom.xml clean install'
}
stage ('archive') {
archiveArtifacts '**/*.jar'
}
stage ('Docker Build') {
// Build and push image with Jenkins' docker-plugin
withDockerRegistry([credentialsId: "fa32f95a-2d3e-4c7b-8f34-11bcc0191d70", url: "https://index.docker.io/v1/"]) {
image = docker.build("your_docker_user_id/mywebapp", "MyAwesomeApp")
image.push()
}
}
stage('docker stop container') {
sh 'docker ps -f name=myContainer -q | xargs --no-run-if-empty docker container stop'
sh 'docker container ls -a -fname=myContainer -q | xargs -r docker container rm'
}
stage ('Docker run') {
image.run("-p 8085:8085 --rm --name myContainer")
}
}
Step #1 - Create Credentials for Docker Hub
Go to your Jenkins where you have installed Docker as well. Go to credentials -->
Click on Global credentials
Click on Add Credentials
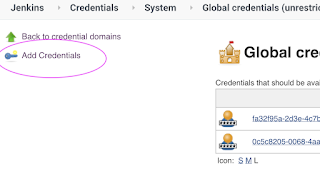
Now Create an entry for Docker Hub credentials
Make sure you take note of the ID as circled below:
Step # 2 - Create a scripted pipeline in Jenkins, name can be anything
Step # 3 - Copy the pipeline code from below
Make sure you change red highlighted values below:
Your docker user id should be updated.
your registry credentials ID from Jenkins from step # 1 should be copied
node {
def image
def mvnHome = tool 'Maven3'
stage ('checkout') {
checkout([$class: 'GitSCM', branches: [[name: '*/master']], doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [], userRemoteConfigs: [[credentialsId: '', url: 'https://bitbucket.org/ananthkannan/myawesomeangularapprepo/']]])
}
stage ('Build') {
sh 'mvn -f MyAwesomeApp/pom.xml clean install'
}
stage ('archive') {
archiveArtifacts '**/*.jar'
}
stage ('Docker Build') {
// Build and push image with Jenkins' docker-plugin
withDockerRegistry([credentialsId: "fa32f95a-2d3e-4c7b-8f34-11bcc0191d70", url: "https://index.docker.io/v1/"]) {
image = docker.build("your_docker_user_id/mywebapp", "MyAwesomeApp")
image.push()
}
}
stage('docker stop container') {
sh 'docker ps -f name=myContainer -q | xargs --no-run-if-empty docker container stop'
sh 'docker container ls -a -fname=myContainer -q | xargs -r docker container rm'
}
stage ('Docker run') {
image.run("-p 8085:8085 --rm --name myContainer")
}
}
Step # 4 - Click on Build to build the pipeline
Once you create the pipeline and changes values per your Docker user id and credentials ID, click on Build Now
Once build is successful, you should see stage view like below:
Steps # 5 - Access Java App which is running inside Docker container
go to browser and enter http://public_dns_name:8085
You should see page like below:
Add credentials 2021
ReplyDeletehttps://docs.cloudbees.com/docs/cloudbees-ci/latest/cloud-secure-guide/injecting-secrets