We
will learn how to automate Docker builds using Jenkins pipelines and Deploy into
AWS EKS - Kubernetes Cluster with help of Kubernetes Continuous Deploy plug-in.
We will use Springboot Microservices based Java application. I have already
created a repo with source code + Dockerfile. The repo also have
Jenkinsfile for automating the following:
- Automating builds using Jenkins
- Automating Docker image creation
- Automating Docker image upload into Docker Hub
- Automating Deployments to Kubernetes Cluster
1. Amazon EKS Cluster is setup and running. Click here to learn how to create Amazon EKS cluster.
4. Docker, Docker pipeline and Kubernetes Continuous Deploy plug-ins are installed in Jenkins
Step #1 -Make sure Jenkins can run Docker builds after validating per pre-requisites
Click on Global credentials
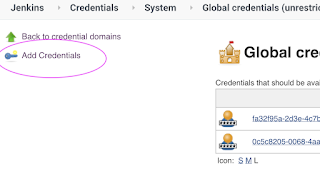
Now Create an entry for your Docker Hub account. Make sure you enter the ID as dockerhub
Step # 4 - Create Maven3 variable under Global tool configuration in Jenkins
By default, clusterrolebinding has system:anonymous set which blocks the cluster access. Execute the following command to set a clusterrole as cluster-admin which will give you the required access.
Make sure you change red highlighted values below as per your settings:
Your docker user id should be updated.
your registry credentials ID from Jenkins from step # 1 should be copied
node ("slave") {
def image
def mvnHome = tool 'Maven3'
stage ('checkout') {
checkout([$class: 'GitSCM', branches: [[name: '*/master']], doGenerateSubmoduleConfigurations: false, extensions: [], submoduleCfg: [], userRemoteConfigs: [[credentialsId: '', url: 'https://bitbucket.org/ananthkannan/myawesomeangularapprepo/']]])
}
stage ('Build') {
sh 'mvn -f MyAwesomeApp/pom.xml clean install'
}
stage ('Docker Build') {
// Build and push image with Jenkins' docker-plugin
withDockerRegistry([credentialsId: "dockerhub", url: "https://index.docker.io/v1/"]) {
image = docker.build("akdevopscoaching/mywebapp", "MyAwesomeApp")
image.push()
}
}
stage ('K8S Deploy') {
kubernetesDeploy(
configs: 'MyAwesomeApp/springboot-lb.yaml',
kubeconfigId: 'K8S',
enableConfigSubstitution: true
)
}
}